· Miler Roque · Herramienta · 2 min lectura
Quicktype, herramienta online para convertir objetos JSON a clases DART (JSON to Dart)
Quicktype es una herramienta online fácil y simple de usar para convertir objetos JSON a Clases en Dart(Flutter) u otros lenguajes.
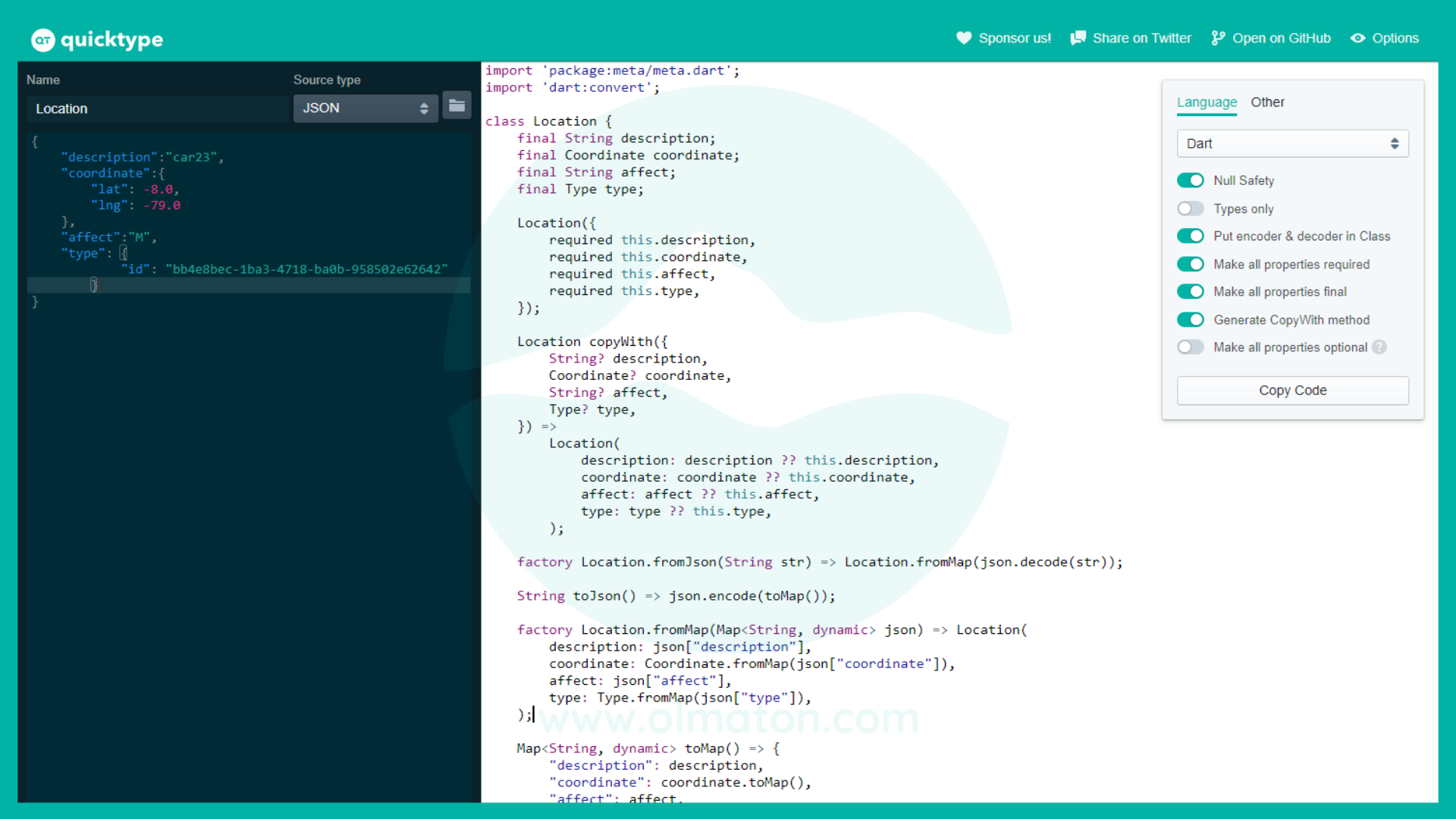
Quicktype es una herramienta online fácil y simple de usar para convertir objetos JSON a Clases en Dart(Flutter) u otros lenguajes. No solo convierte a clases, sino que genera funciones como: toJson, toMap, copyWith y más. Disponible en: https://app.quicktype.io
Ejemplo de objeto JSON:
{
"description": "car23",
"coordinate": {
"lat": -8.0,
"lng": -79.0
},
"affect": "M",
"type": {
"id": "bb4e8bec-1ba3-4718-ba0b-958502e62642"
}
}
Ejemplo de código en Dart:
import 'dart:convert';
class Location {
final String description;
final Coordinate coordinate;
final String affect;
final Type type;
Location({
required this.description,
required this.coordinate,
required this.affect,
required this.type,
});
Location copyWith({
String? description,
Coordinate? coordinate,
String? affect,
Type? type,
}) =>
Location(
description: description ?? this.description,
coordinate: coordinate ?? this.coordinate,
affect: affect ?? this.affect,
type: type ?? this.type,
);
factory Location.fromJson(String str) => Location.fromMap(json.decode(str));
String toJson() => json.encode(toMap());
factory Location.fromMap(Map<String, dynamic> json) => Location(
description: json["description"],
coordinate: Coordinate.fromMap(json["coordinate"]),
affect: json["affect"],
type: Type.fromMap(json["type"]),
);
Map<String, dynamic> toMap() => {
"description": description,
"coordinate": coordinate.toMap(),
"affect": affect,
"type": type.toMap(),
};
}
class Coordinate {
final int lat;
final int lng;
Coordinate({
required this.lat,
required this.lng,
});
Coordinate copyWith({
int? lat,
int? lng,
}) =>
Coordinate(
lat: lat ?? this.lat,
lng: lng ?? this.lng,
);
factory Coordinate.fromJson(String str) => Coordinate.fromMap(json.decode(str));
String toJson() => json.encode(toMap());
factory Coordinate.fromMap(Map<String, dynamic> json) => Coordinate(
lat: json["lat"],
lng: json["lng"],
);
Map<String, dynamic> toMap() => {
"lat": lat,
"lng": lng,
};
}
class Type {
final String id;
Type({
required this.id,
});
Type copyWith({
String? id,
}) =>
Type(
id: id ?? this.id,
);
factory Type.fromJson(String str) => Type.fromMap(json.decode(str));
String toJson() => json.encode(toMap());
factory Type.fromMap(Map<String, dynamic> json) => Type(
id: json["id"],
);
Map<String, dynamic> toMap() => {
"id": id,
};
}